회사에서 동료들과 동호회 활동을 하고 있습니다.
각 맴버의 활동 정보를 slack 챗봇을 만들어 알림 처리를 하고 있었어요.
slack 알림은 보고자 하는 내용을 최소한으로 가독성 있게 보려는 컨셉으로 접근하다보니 한계가 있더라구요,
그래서 웹에서 조회 해보려고 해요.
단, 가장 빠르고 편하게, 최소한의 노력만으로 보고자 하는 화면을 만들어 내야 합니다. 일을 해야하거든요.
오늘은 Postgresql에서 Node.js 를 사용하여 빠르게 맴버목록을 조회 해볼께요
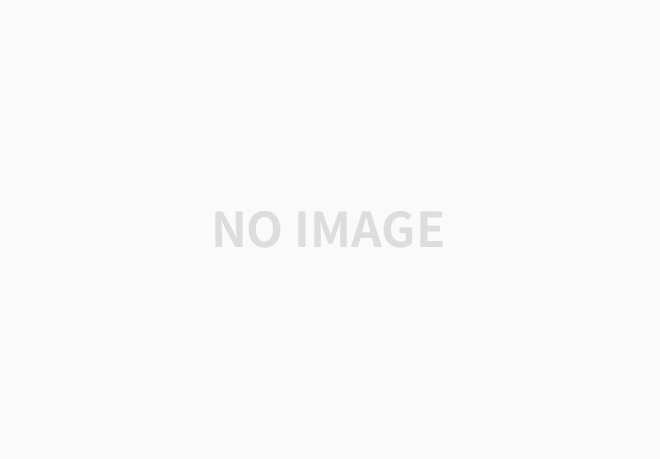
project 폴더 생성
mkdir pg-express-app
cd pg-express-app
package.json 파일 생성
npm init -y
pg 패키지 설치
pg는 Node.js 애플리케이션에서 PostgreSQL 데이터베이스와 상호작용하기 위한 공식 패키지입니다. 이 패키지를 사용하면 PostgreSQL 데이터베이스에 연결하고, SQL 쿼리를 실행하며, 데이터베이스에서 결과를 가져올 수 있습니다.
npm install pg
express 패키지 설치
Express는 Node.js를 위한 웹 애플리케이션 프레임워크로, 서버 사이드 애플리케이션을 쉽게 구축할 수 있도록 도와줍니다. 간단하고 유연한 구조로, 다양한 미들웨어를 사용하여 HTTP 요청 및 응답을 쉽게 처리할 수 있습니다.
npm install express
server.js 파일 생성
2024.06.05 - [Language] - [Node.js] .env 파일로 환경변수를 관리 하기
pg-express-app/
├── node_modules/
├── public/
│ └── index.html
├── server.js
├── package.json
└── package-lock.json
const express = require('express');
const path = require('path');
const { Client } = require('pg');
const app = express();
const port = 3000;
//POSTGRESQL 접속정보
const client = new Client({
user: 'fubarvis',
host: 'localhost',
database: 'fubarvis',
password: 'fubarvis',
port: 5432,
});
client.connect();
app.use(express.static(path.join(__dirname, 'public')));
// 시간조회
app.get('/get-time', (req, res) => {
client.query('SELECT NOW()', (err, dbRes) => {
if (err) {
console.error(err);
res.status(500).send('Database error');
} else {
res.json(dbRes.rows[0]);
}
});
});
// 멤버조회
app.get('/get-members', (req, res) => {
client.query('SELECT * FROM MEMBER', (err, dbRes) => {
if (err) {
console.error(err);
res.status(500).send('Database error');
} else {
res.json(dbRes.rows);
}
});
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
index.html 파일 생성
pg-express-app/
├── node_modules/
├── public/
│ └── index.html
├── server.js
├── package.json
└── package-lock.json
<html>
<head>
<title>FUBABAZ</title>
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet">
</head>
<body class="container">
<h1 class="mt-5 mb-3">FUBABAZ MEMBERS</h1>
<button id="fetchButton" class="btn btn-primary mb-3">FETCH</button>
<div id="members"></div>
<script>
function fetchData() {
document.getElementById('members').innerHTML = '';
fetch('/get-time')
.then(response => response.json())
.then(data => {
const timeResultElement = document.createElement('p');
timeResultElement.classList.add('lead');
timeResultElement.innerText = 'Fetch Time: ' + data.now;
document.getElementById('members').appendChild(timeResultElement);
})
.catch(error => console.error('Error fetching time data:', error));
fetch('/get-members')
.then(response => response.json())
.then(data => {
const table = document.createElement('table');
table.classList.add('table');
const thead = document.createElement('thead');
thead.innerHTML = `
<tr>
<th>Image</th>
<th>Github ID</th>
<th>Baekjoon ID</th>
<th>Level</th>
<th>Blog ID</th>
<th>Blog URL</th>
</tr>`;
table.appendChild(thead);
const tbody = document.createElement('tbody');
data.forEach(member => {
const row = document.createElement('tr');
row.innerHTML = `
<td><img src="${member.image}" style='max-width: 25px;' alt="Member Image"></td>
<td>${member.github_id}</td>
<td>${member.baekjoon_id}</td>
<td>${member.lev}</td>
<td>${member.blog_id}</td>
<td>${member.blog_url ? `<a href="${member.blog_url}" target="_blank">${member.blog_url}</a>` : '-'}</td> `;
tbody.appendChild(row);
});
table.appendChild(tbody);
document.getElementById('members').appendChild(table);
})
.catch(error => console.error('Error fetching member data:', error));
}
document.getElementById('fetchButton').addEventListener('click', fetchData);
</script>
</body>
</html>
server 실행
실행완료후 http://localhost:3000 접속
node server.js
'Language' 카테고리의 다른 글
[Node.js] .env 파일로 환경변수를 관리 하기 (0) | 2024.06.05 |
---|---|
[Language] C언어, C++, JAVA, Python의 차이점 (0) | 2022.09.03 |